Meta Box WordPress là gì?
Meta Box WordPress là một hộp có thể kéo thả được hiển thị trên màn hình chỉnh sửa bài đăng. Mục đích của nó là cho phép người dùng chọn hoặc nhập thông tin ngoài nội dung chính của bài đăng.
Thông thường có hai loại Meta Box:
- Metadata (i.e. custom fields)
- Taxonomy terms
Tất nhiên, có những Meta Box khác, nhưng hai loại trên là được sử dụng phổ biến nhất. Với mục đích của hướng dẫn này, bạn sẽ học cách tạo một Custom Meta Box WordPress.
Post Metadata là gì?
Post metadata là dữ liệu được lưu trong bảng wp_postmeta với 4 fields dữ liệu:
meta_id
: unique ID- post_id: lưu post ID mà metadata đính kèm theo post này.
- meta_key: là key xác định dữ liệu
- meta_value: là giá trị của metadata.
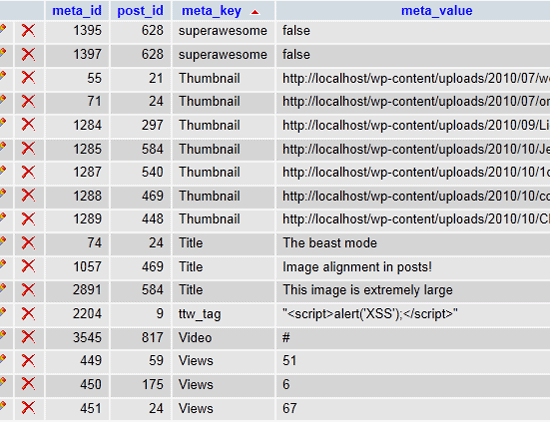
Lưu ý: Một điều cần lưu ý là một key meta có thể có nhiều giá trị meta.
Để làm việc với post metadata chúng ta thường sử dụng các function
- add_post_meta(): Thêm post metadata.
- update_post_meta(): Cập nhập post metadata.
- delete_post_meta(): Xóa post metadata.
- get_post_meta(): Lấy giá trị post metadata.
Tạo một Custom Post Meta Box
Để xuất hiện post meta box của bạn cần sử dụng hooks load-post.php
và load-post-new.php.
Dưới đây là code tạo một Custom Post Meta Box
/* Fire our meta box setup function on the post editor screen. */
add_action( 'load-post.php', 'smashing_post_meta_boxes_setup' );
add_action( 'load-post-new.php', 'smashing_post_meta_boxes_setup' );
/* Meta box setup function. */
function smashing_post_meta_boxes_setup()
{
/* Add meta boxes on the 'add_meta_boxes' hook. */
add_action('add_meta_boxes', 'smashing_add_post_meta_boxes');
}
/* Create one or more meta boxes to be displayed on the post editor screen. */
function smashing_add_post_meta_boxes()
{
add_meta_box(
'smashing-post-class', // Unique ID
esc_html__('Post Class', 'example'), // Title
'smashing_post_class_meta_box', // Callback function
'post', // Admin page (or post type)
'side', // Context
'default' // Priority
);
}
/* Display the post meta box. */
function smashing_post_class_meta_box($post)
{ ?>
<?php wp_nonce_field(basename(__FILE__), 'smashing_post_class_nonce'); ?>
<p>
<label for="smashing-post-class"><?php _e("Add a custom CSS class, which will be applied to WordPress' post class.", 'example'); ?></label>
<br />
<input class="widefat" type="text" name="smashing-post-class" id="smashing-post-class" value="<?php echo esc_attr(get_post_meta($post->ID, 'smashing_post_class', true)); ?>" size="30" />
</p>
<?php }
Kết quả nhận được khi bạn vào edit post
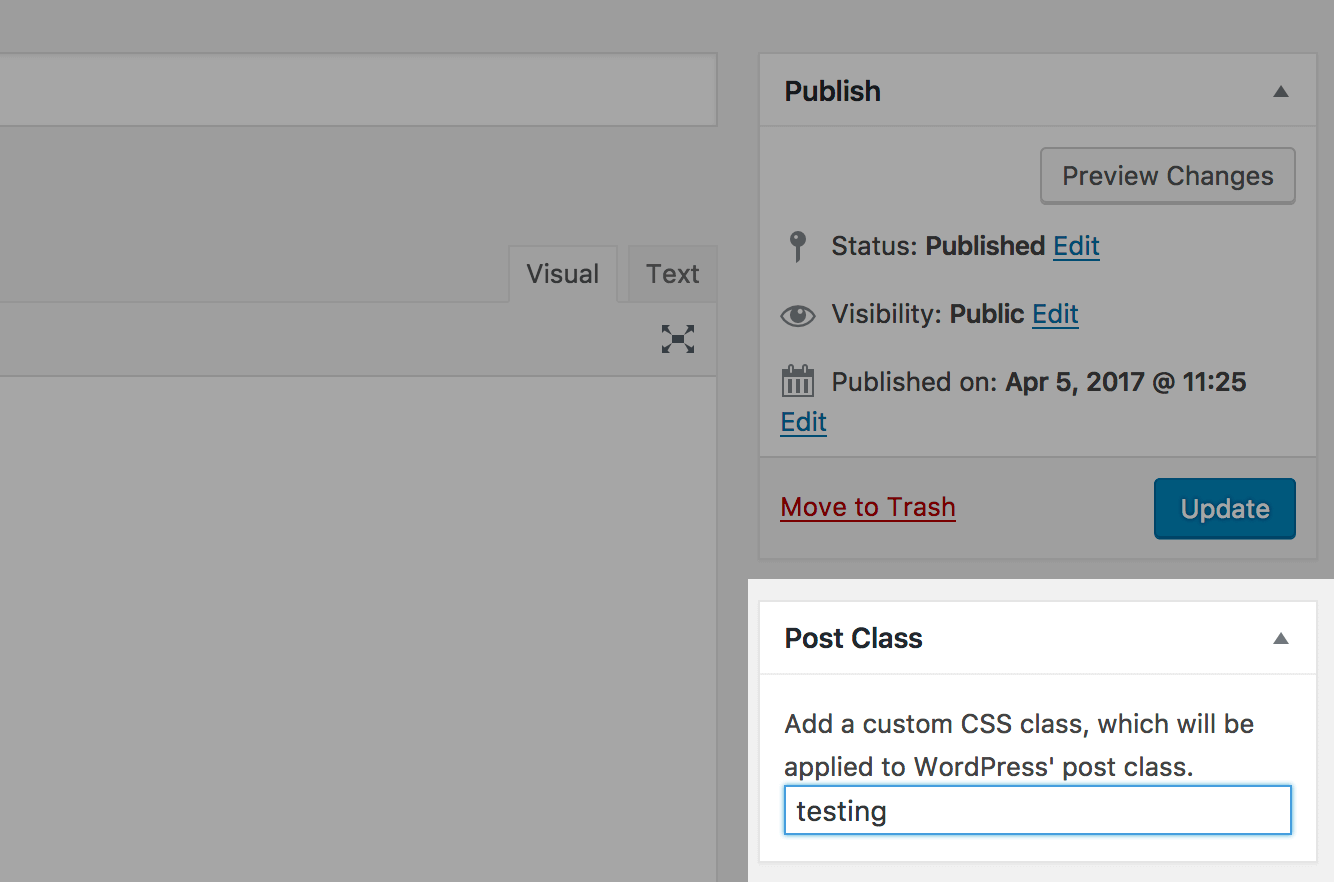
Lưu giá trị của Custom Post Meta Box
Để lưu được bạn cần dùng hook 'save_post', ta sửa lại function smashing_post_meta_boxes_setup, như sau.
/* Meta box setup function. */
function smashing_post_meta_boxes_setup() {
/* Add meta boxes on the 'add_meta_boxes' hook. */
add_action( 'add_meta_boxes', 'smashing_add_post_meta_boxes' );
/* Save post meta on the 'save_post' hook. */
add_action( 'save_post', 'smashing_save_post_class_meta', 10, 2 );
}
Và function xử lý lưu dữ liệu của post meta box:
/* Save the meta box’s post metadata. */
function smashing_save_post_class_meta($post_id, $post)
{
/* Verify the nonce before proceeding. */
if (!isset($_POST['smashing_post_class_nonce']) || !wp_verify_nonce($_POST['smashing_post_class_nonce'], basename(__FILE__)))
return $post_id;
/* Get the post type object. */
$post_type = get_post_type_object($post->post_type);
/* Check if the current user has permission to edit the post. */
if (!current_user_can($post_type->cap->edit_post, $post_id))
return $post_id;
/* Get the posted data and sanitize it for use as an HTML class. */
$new_meta_value = (isset($_POST['smashing-post-class']) ? sanitize_html_class($_POST['smashing-post-class']) : ’);
/* Get the meta key. */
$meta_key = 'smashing_post_class';
/* Get the meta value of the custom field key. */
$meta_value = get_post_meta($post_id, $meta_key, true);
/* If a new meta value was added and there was no previous value, add it. */
if ($new_meta_value && '' == $meta_value)
add_post_meta($post_id, $meta_key, $new_meta_value, true);
/* If the new meta value does not match the old value, update it. */
elseif ($new_meta_value && $new_meta_value != $meta_value)
update_post_meta($post_id, $meta_key, $new_meta_value);
/* If there is no new meta value but an old value exists, delete it. */
elseif ('' == $new_meta_value && $meta_value)
delete_post_meta($post_id, $meta_key, $meta_value);
}
Hiển thị dữ liệu Custom Post Meta Box
Ví dụ : Đoạn code sau thêm class bài (nếu có) từ Custom Post Meta Box của bạn.
/* Filter the post class hook with our custom post class function. */
add_filter( 'post_class', 'smashing_post_class' );
function smashing_post_class( $classes ) {
/* Get the current post ID. */
$post_id = get_the_ID();
/* If we have a post ID, proceed. */
if ( !empty( $post_id ) ) {
/* Get the custom post class. */
$post_class = get_post_meta( $post_id, 'smashing_post_class', true );
/* If a post class was input, sanitize it and add it to the post class array. */
if ( !empty( $post_class ) )
$classes[] = sanitize_html_class( $post_class );
}
return $classes;
}